Raspberry Pi Pico on Mac with C++ — part 1: Required softwares
Software requirements for Pico development.
The first goal of my offseason project is to make the synth work with any MIDI controller. For this I need to build a little device that turns MIDI to control voltages—a MIDI to CV converter. Even though last year I started making stuff for STM32, due to chip shortage for an unpredictable period of time I opted for a board that is still available🤞: the Raspberry Pi Pico.
This series is about how to get started with the Pico board with C++ on a Mac. A couple of assumptions:
- You're using an Intel Mac. This might not work on M1 chip Mac's
- You know what a package manager is
- You understand the basics of how C/C++ works. The Cherno has an amazing series about it on YouTube if you needed a quick refresh
- You know what a terminal and a shell is and how to edit your profile
- You keep your code in a directory called
~/Code
. If not, just watch out with paths in terminal commands - You have two Pico boards and a bunch of jumper cables
Please make sure you got these before getting started. In the first part we're going to install the required softwares.
To be able to get started with the Raspberry Pi Pico – or any other embedded development FWIW – a bunch of software is needed even just to make the simplest thing, like blinking an LED.
XCode
Yeah, we need xcode so go to the App store on your Mac and install it. Wait 2 weeks and boom you have xcode.
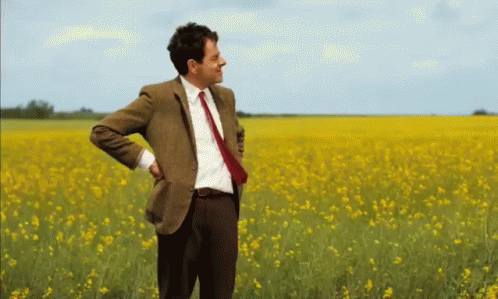
VSCode
I'm sure you have VSCode already but if you don't, then get it from here. Make sure you install the command line prompt code
too and download the following extensions:
- C++
- Cortex debug (by marus25)
- CMake (by Microsoft) plugins.
XCode home
VSCode home
Homebrew
Homebrew is a package manager which we'll need to install some stuff. If you don't have it run this in your terminal:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
Homebrew home
CMake
We'll need CMake to build our C/C++ programs. It's a tool that generates makefiles and makes the whole build process super-simple.
brew install cmake
cmake --version
CMake home
GNU ARM embedded toolchain (homebrew or manual installation)
GNU ARM embedded toolchain is a bunch of programs that'll cross compile C/C++ code for ARM microprocessors. There are two ways to install it:
Option 1—using homebrew (this is simpler):
brew tap ArmMbed/homebrew-formulae
brew install arm-none-eabi-gcc
Option 2—download, extract and add it to your path (here xxxxxxx
might change based on the latest version of the toolchain)
- Download the GNU ARM Embedded Toolchain tar file from here
- Double click to unzip it
- Create a folder for it:
mkdir /usr/local/gcc_arm
- Move the unzipped folder to it:
mv ~/Downloads/gcc-arm-none-eabi-xxxxxxx /usr/local/gcc_arm/
- Add the
bin
folder to your path in your profile:export PATH="$PATH:/usr/local/gcc_arm/gcc-arm-none-eabi-xxxxxxx/bin/"
arm-none-eabi-gcc --version
GNU ARM Embedded Toolchain home
OpenOCD
No it's not that OC D. It's a host tool that enables debugging by managing the communication between the microcontroller and the debugger (OCD stands for On Chip Debugging). Run these to get it:
brew install libtool automake texinfo wget gcc pkg-config libusb
export PATH="/opt/homebrew/opt/texinfo/bin:$PATH"
cd ~/Code
git clone https://github.com/raspberrypi/openocd.git \
--branch picoprobe --depth=1
cd openocd
./bootstrap
./configure --enable-picoprobe --disable-werror
make -j4
sudo make install
OpenOCD home
Minicom (optional)
It's a cool little utility that will let us use printf
commands to output stuff on the computer's screen. You use this more than you think during embedded development.
brew install minicom
😅 Pfhew, that's all the software we need.
In the next post of the series, we make a super-simple program to blink an LED.